일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Python
- langchain
- 파이썬
- 판다스
- 자연어분석
- fastapi #python웹개발
- fastapi #파이썬웹개발
- 비지도학습
- NLP
- 사이킷런
- 판다스 데이터정렬
- pytorch
- fastapi
- 파이토치
- pandas
- 파이토치기본
- 랭체인
- OpenAIAPI
- sklearn
- 머신러닝
- deeplearning
- MachineLearning
- programmablesearchengine
- 챗gpt
- python 정렬
- 파이썬웹개발
- HTML
- 딥러닝
- konlpy
- chatGPT
- Today
- Total
Data Navigator
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기5 - CRUD 구현 하기 본문
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기5
- CRUD 구현 하기 -
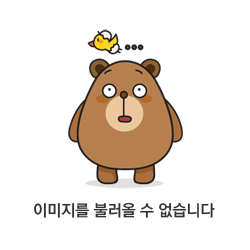
지금까지 만든 todo 어플리케이션에 아이템 변경 삭제를 할 수 있도록 기능을 추가한다.
1. update 기능 추가하기
1) update 라우트의 요청 바디용 모델을 model.py에 추가한다.
class TodoItem(BaseModel):
item : str
class Config:
schema_extra = {
"example" : {
"item" : "Read the next chapter of the book."
}
}
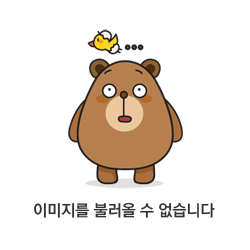
2) todo.py에 todo 변경을 위한 라우트를 추가한다.
model에 새로 정의한 TodoItem 을 import 한다.
# todo.py
from fastapi import APIRouter, Path
from model import Todo, TodoItem # TodoItem 추가
todo_router = APIRouter()
todo_list = []
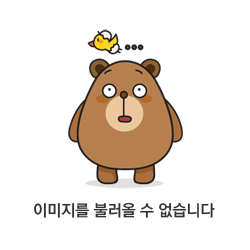
3) todo.py에 update 기능을 추가한다.
@todo_router.put("/todo/{todo_id}")
async def update_todo(todo_data: TodoItem, todo_id: int = Path(..., title="The ID of the todo to be updated")) -> dict:
for todo in todo_list:
if todo.id == todo_id:
return {
"messsage" : "Todo updated successfully."
}
return {
"message" : "Todo with supplied ID doesn't exist."
}
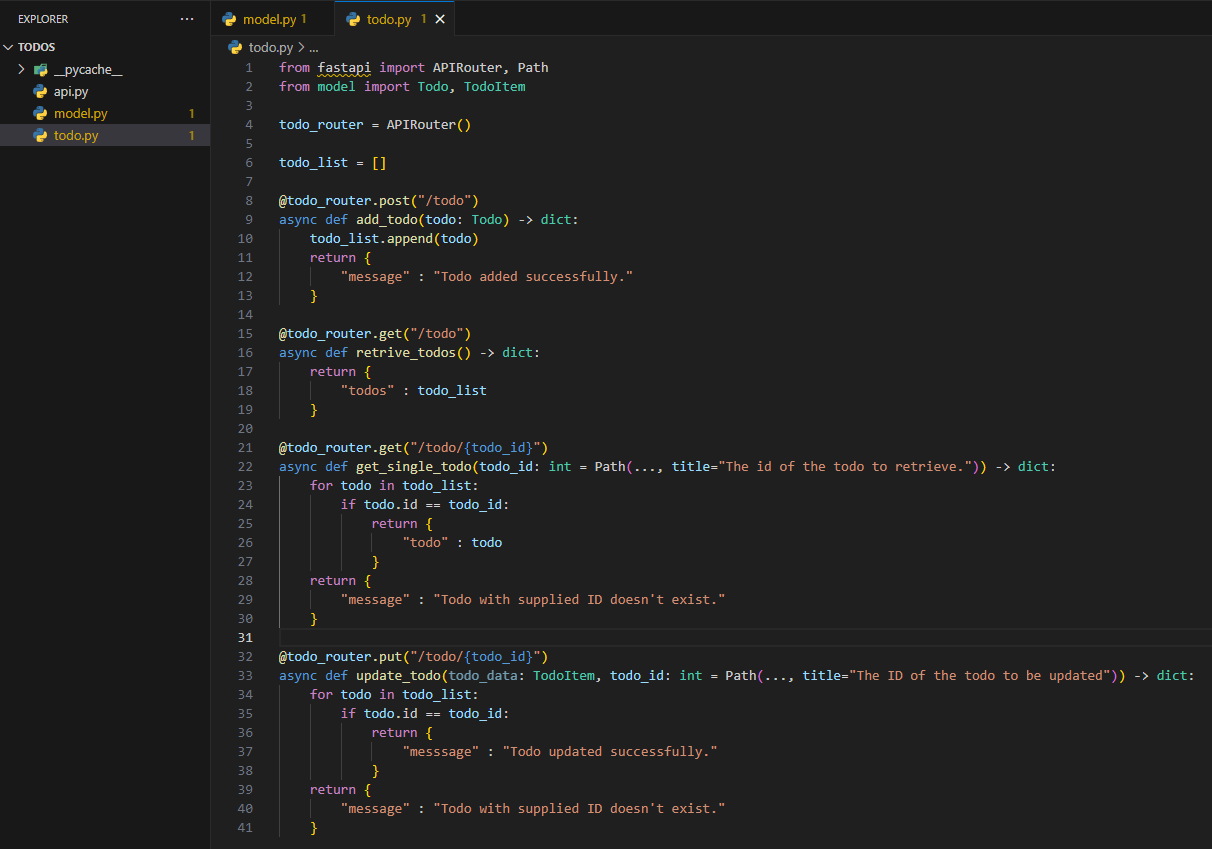
4) 새로 추가한 라우트를 테스트하기 위해 신규 todo 아이템 추가
서버를 실행하고 localhost:8000/docs로 접속해 post /todo 부분의 try it out으로 새 아이템을 추가한다.
{
"id": 1,
"item": "새 라우트 테스트"
}
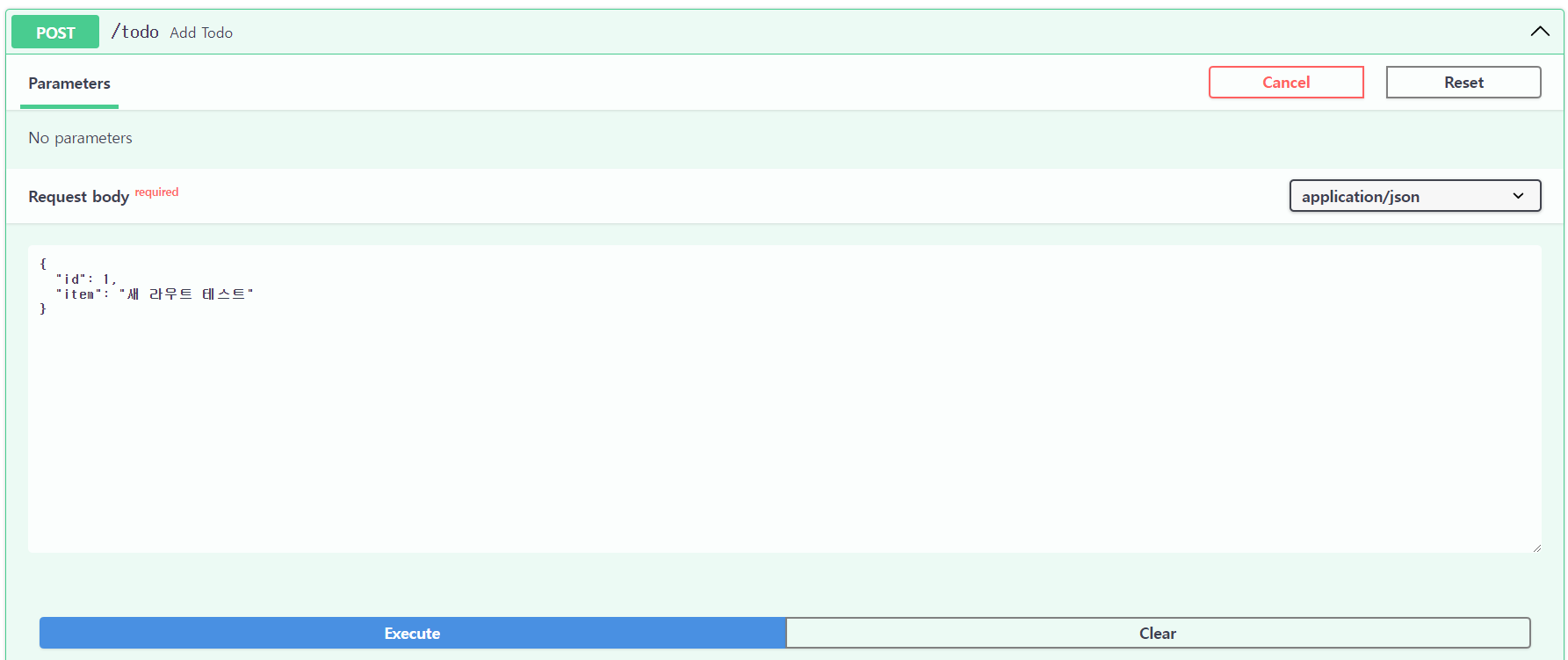
excute를 누르고 server response에 200코드와 "message": "Todo added successfully."가 뜨는지 확인한다.

5) 추가한 아이템 확인
localhost:8000/docs의 get /todo/{todo_id} Get Single Todo 부분에서 try it out을 누르고 todo_id에 1을 입력한 후 excute를 눌러 위에서 입력한 { "id": 1, "item": "새 라우트 테스트"} 가 정상적으로 출력되는지 확인한다.
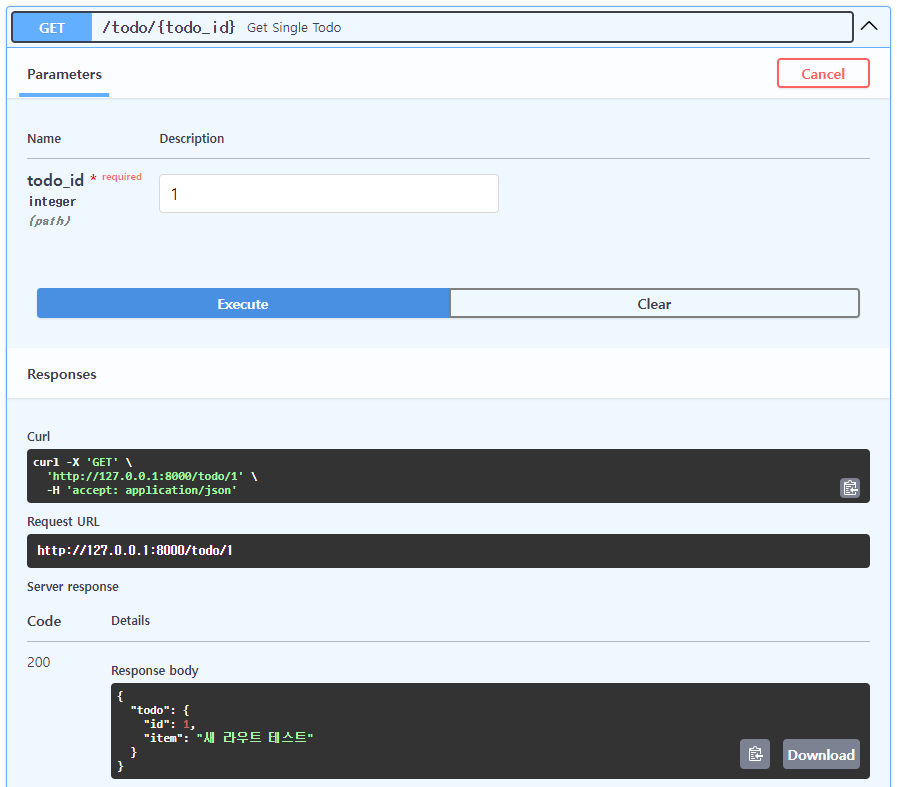
6) id 1번의 내용 update하기
localhost:8000/docs의 put /todo/{todo_id} Update Todo의 try it out을 누르고 todo_id에 1, Request body의 "item" : "string"부분의 string에 새로 업데이트할 내용(새 라우트 테스트 업데이트)으로 바꾸고 excute를 누른다.
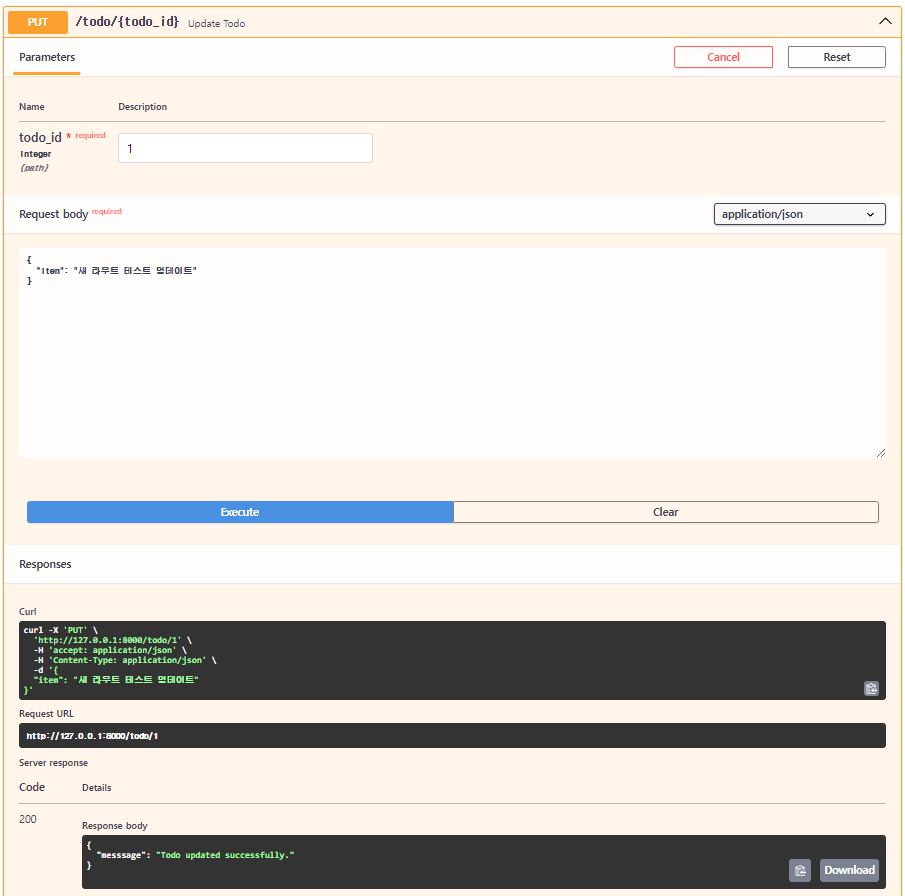
7) 업데이트 된 내용 확인하기
localhost:8000/docs의 get /todo/{todo_id} Get Single Todo 부분에서 try it out을 누르고 todo_id에 1을 입력한 후 excute를 눌러 위에서 업데이트한 { "id": 1, "item": "새 라우트 테스트 업데이트"} 가 정상적으로 출력되는지 확인한다.정상적으로 출력되었다면 추가한 업데이트 라우트가 정상적으로 잘 작동하는 것이다.
2. delete 기능 추가하기
1) todo.py에서 삭제를 위한 delete 라우트를 추가한다.
# todo.py
@todo_router.delete("/todo/{todo_id}")
async def delete_single_todo(todo_id: int) -> dict:
for index in range(len(todo_list)):
todo = todo_list[index]
if todo.id == todo_id:
todo_list.pop(index)
return {
"message" : "Todo deleted successfully."
}
return {
"message" : "Todo with supplied ID doesn't exist."
}
@todo_router.delete("/todo")
async def delete_all_todo() -> dict:
todo_list.clear()
return {
"message" : "Todos deleted successfully."
}
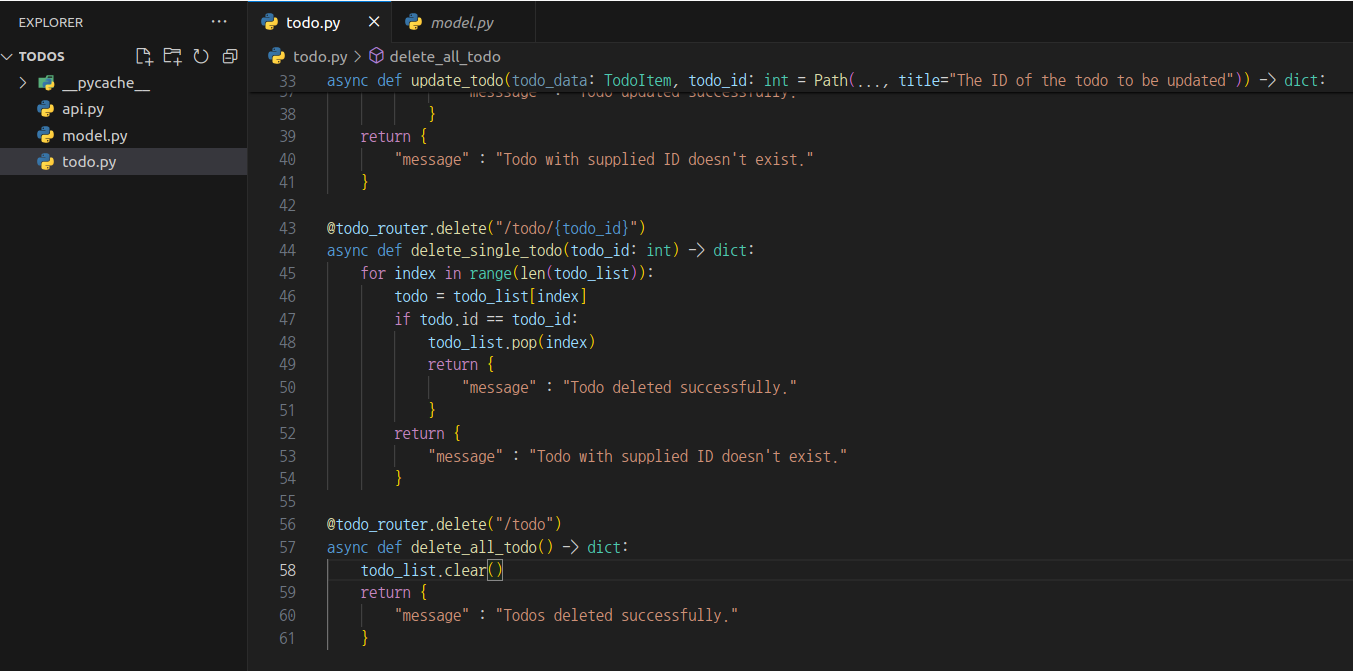
2) delete 기능 테스트하기위해 서버를 실행하고 localhost:8000/docs로 접속해 todo item을 추가한다.
post /todo Add Todo 의 드롭다운 메뉴를 눌러 Try it out 을 누르고 Request body에 나오는 내용중 item의 value를 삭제 테스트로 변경한다.
{
"id": 1,
"item": "삭제테스트!"
}
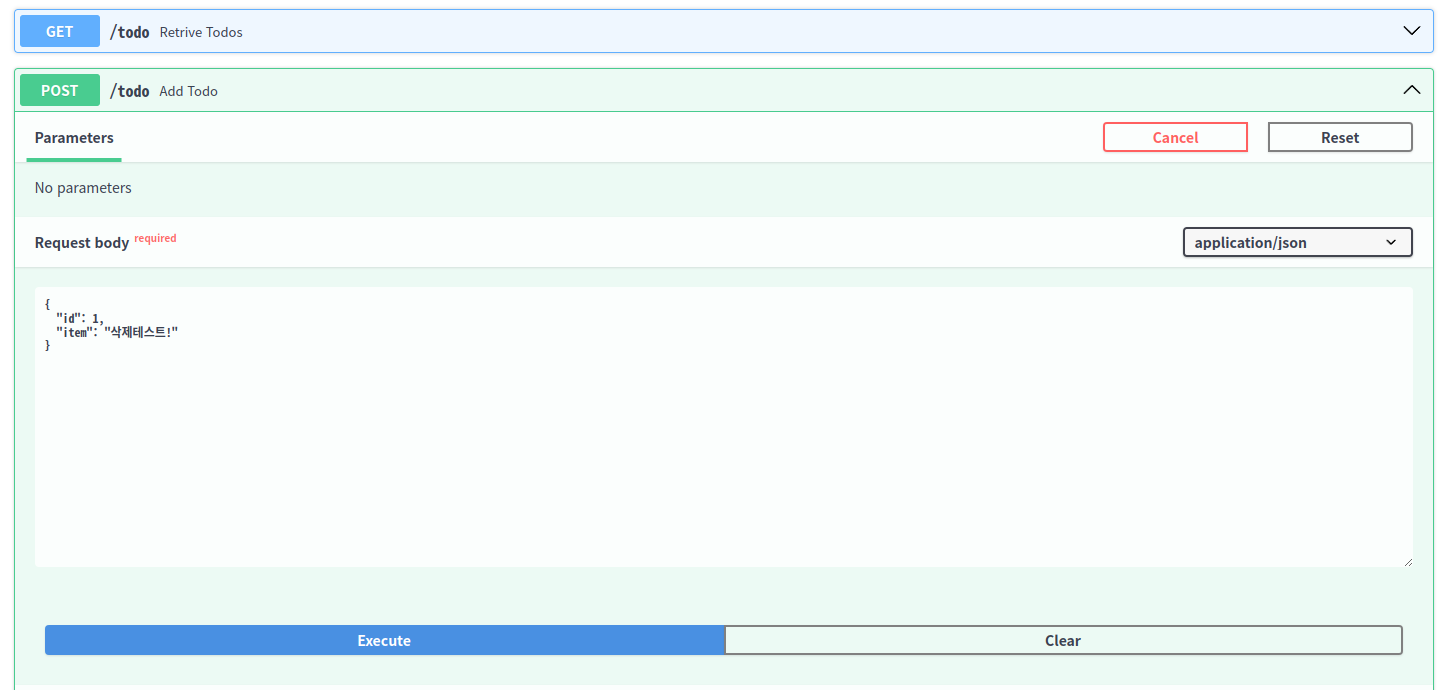
입력 후 excute를 누른뒤 Responses 부분에서 Code 200 과 Response body에 "message" : "Todo added successfully"를 확인한다.
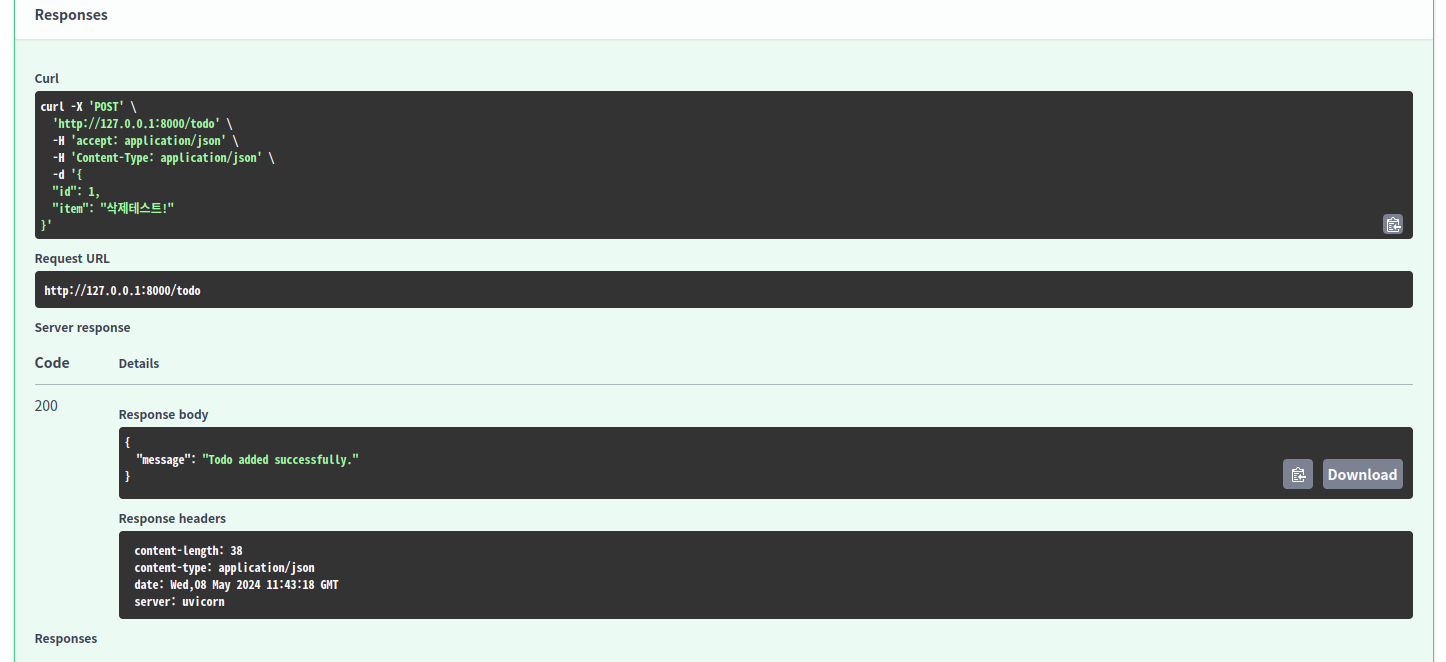
3) 입력된 내용을 확인하기 위해 GET /todo/{todo_id} 드롭다운을 누르고 Try it out 을 누른다.
id 창에 1을 넣고 excute를 누른다.
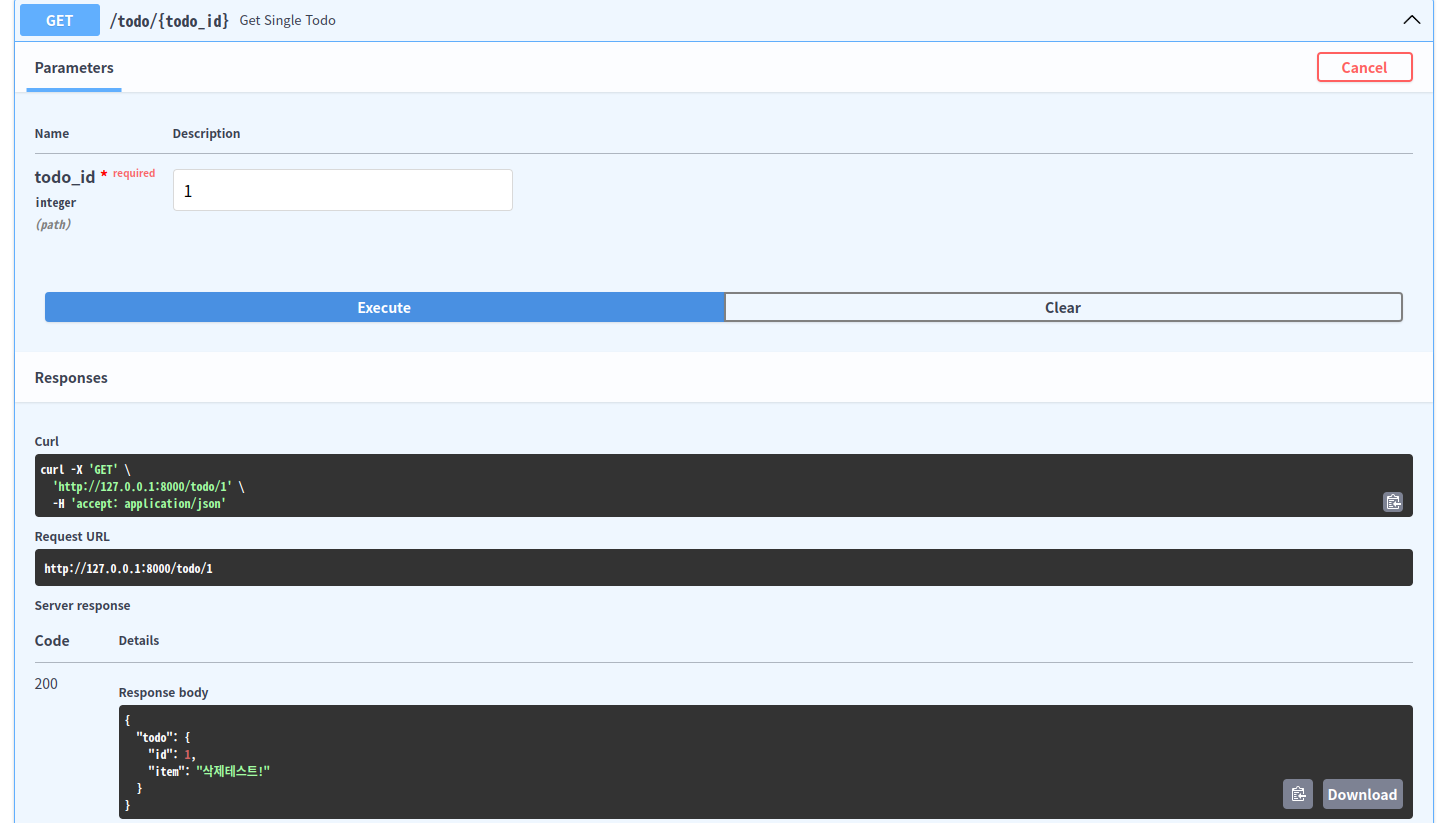
Code 200과 Response body에 위에서 입력한 id : 1 과 item : "삭제테스트!"가 정상적으로 조회 되는지 확인한다.
4) 입력했던 데이터를 삭제해보자.
DELETE /todo/{todo_id} Delete Single Todo 에서 Try it out 을 누르고 Todo_id에 1을 입력 후 excute를 누른다.
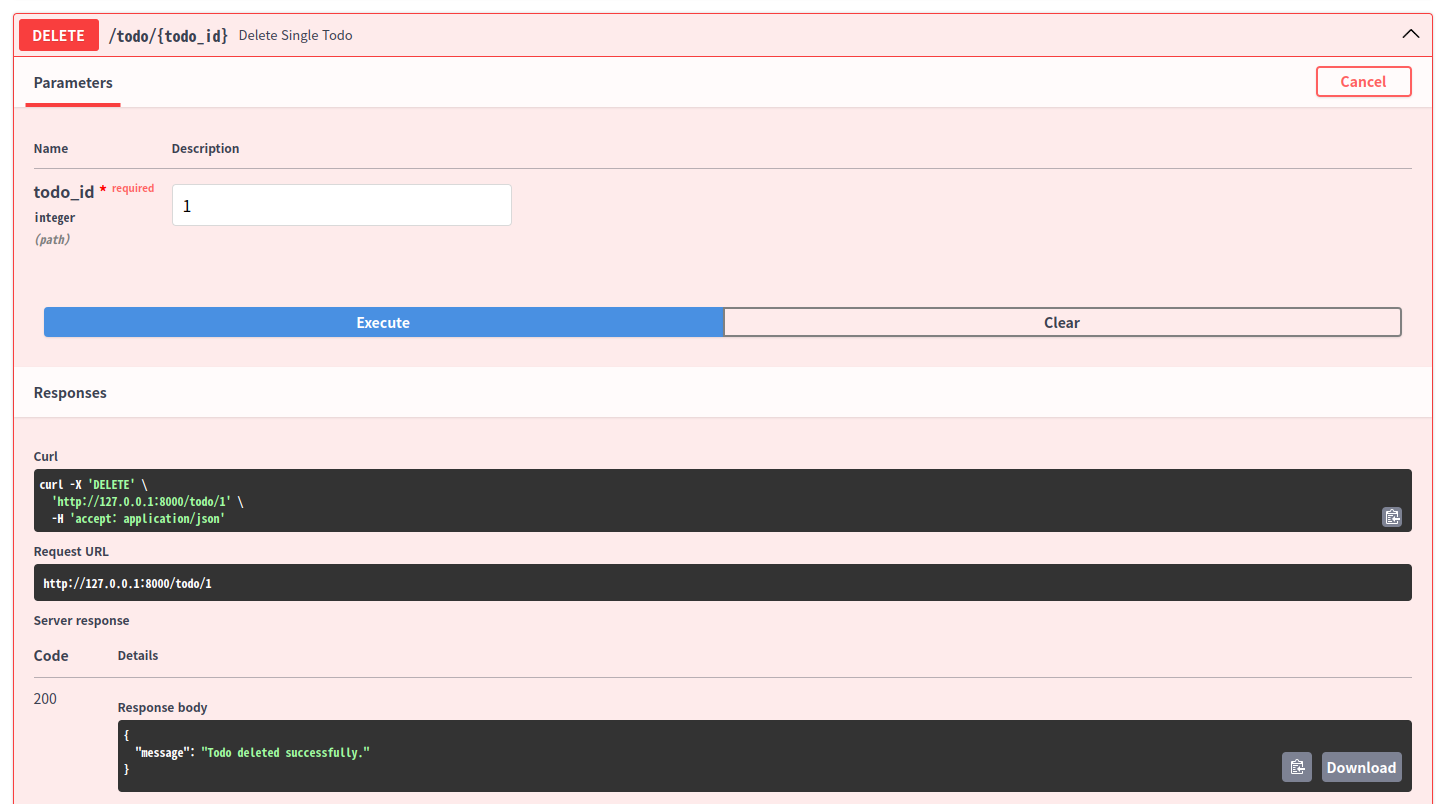
Code 200과 Response body에 "message" : "Todo deleted successfully."가 출력되면 정상이다.
5) 삭제가 정상적으로 되었는지 GET /todo/{todo_id} 으로 다시 가서 드롭다운을 누르고 clear를 눌러 초기화 한 후 Try it out 을 누른다. id 창에 1을 넣고 excute를 누른다.
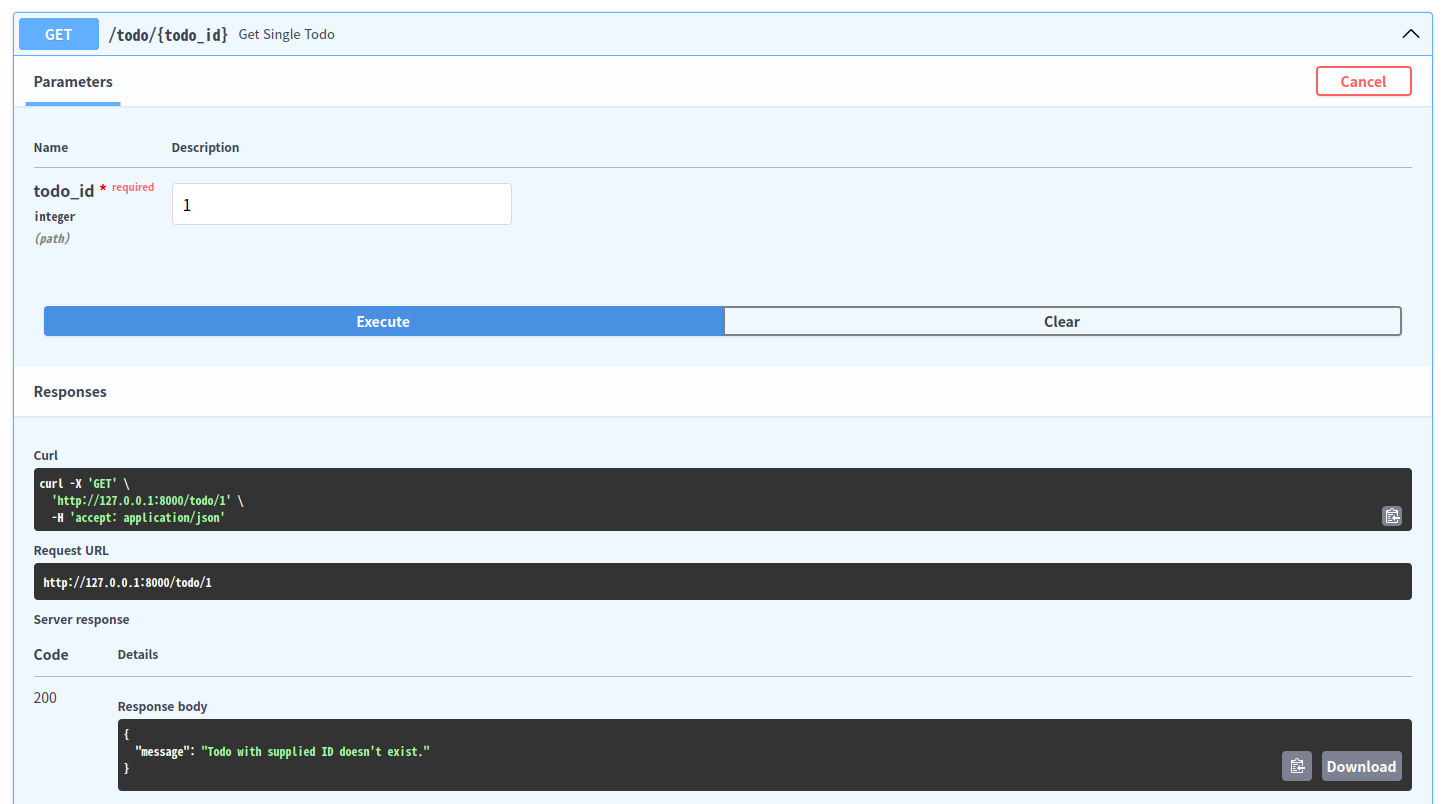
Code 200과 Response body에 message" : "Todo with supplied ID doesn't exist."가 출력되면 성공!!
출처: 아데시나, 압둘라지즈 압둘라지즈. FastAPI를 사용한 파이썬 웹 개발. 번역 김완섭. 한빛미디어, 2023
'FastAPI' 카테고리의 다른 글
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기7 - http 예외처리하기 (0) | 2024.05.09 |
---|---|
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기6 - todo 목록 반환 모델 만들기 (0) | 2024.05.08 |
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기4 - 경로 매개변수와 쿼리 매개변수 (0) | 2024.05.04 |
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기3 - pydantic 으로 유효성 검사하기 (0) | 2024.05.03 |
FastAPI 설치 환경 세팅 및 간단한 테스트 웹 만들기2 - Routing (0) | 2024.05.03 |